There might be an occasion where you’d like to have your WordPress posts be displayed in columns within a Bootstrap “row” like the image below.
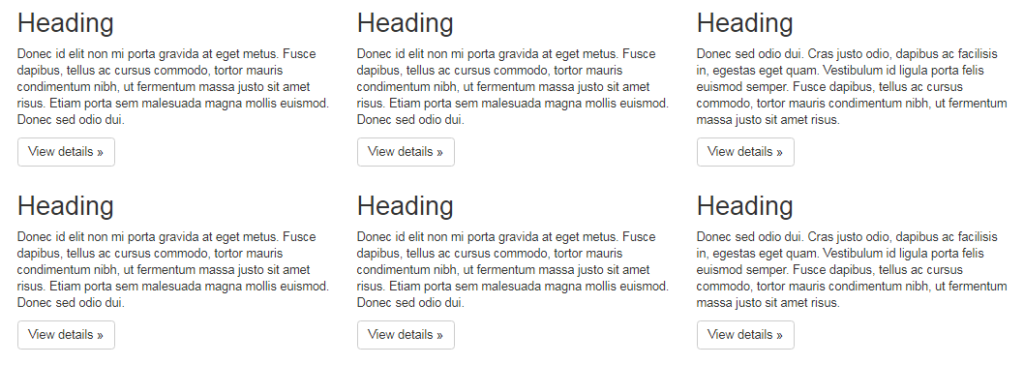
You can do it whether it is in columns of two, three, or more.
Simply copy the sample PHP code below to generate the same column and row layout in your WordPress loop. The code below displays three posts as columns per row. Then it loops the process until all posts are accounted for.
<?php
/* Your loop args */
$args = array(
'post_type' => 'post',
'post_status' => 'publish',
'orderby' => 'date',
'order' => 'DESC',
'posts_per_page' => -1
);
/* New loop */
$post_loop = new WP_Query( $args );
if ( $post_loop->have_posts() ) :
/* start of column count in a row */
$i = 1;
?>
<div class="row">
<?php while ( $post_loop->have_posts() ) : $post_loop->the_post(); ?>
<!-- this is the post in Bootstrap columns. change the class value when necessary -->
<div class="col-md-4">
<div class="blog-post">
<div class="blog-post-details">
<h4><a href="<?php the_permalink();?>"><?php the_title();?></a></h4>
<div class="post-excerpt"><?php the_excerpt();?></div>
<a class="post-link" href="<?php the_permalink();?>">View details</a>
</div>
</div>
</div>
<?php if( $i == 3 ){ /* change this index number to your desired column count which will be displayed per row */ ?>
</div>
<div class="row">
<?php $i = 0;
}
$i++;
?>
<?php endwhile; ?>
</div>
<?php endif; wp_reset_postdata(); ?>
You can change the number of columns per row by changing the index count at Line 31. If you do that, don’t forget to change the Bootstrap class value at Line 22.